Cross Site Scripting (XSS): Common Vulnerabilities and How to Fix Them
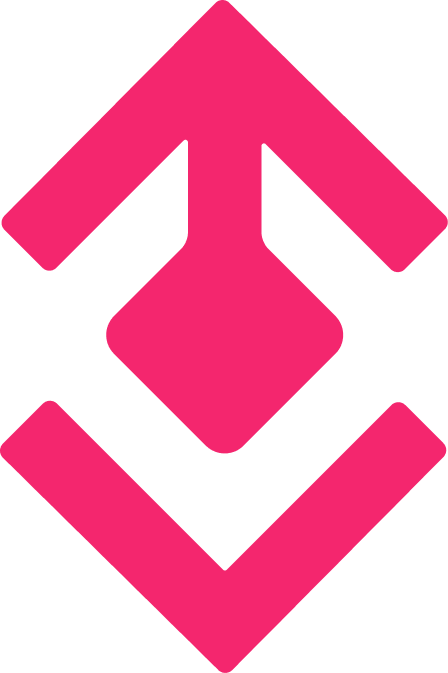
Cross Site Scripting (XSS) remains one of the top 3 most critical web application security risks according to OWASP. XSS attacks continue to plague web applications and lead to data breaches and compromised user accounts, despite being a prominent vulnerability for over two decades.
Web applications become vulnerable to cross-site scripting when they fail to verify or encode user input properly. Attackers can inject malicious scripts into web pages that other users view. These attacks range from stealing session cookies to taking complete control of user accounts. The concept might seem straightforward, but XSS prevention needs a thorough understanding of attack vectors and implementation of reliable security measures.
This detailed guide will help you understand common XSS vulnerabilities, see practical attack scenarios, and learn prevention strategies to secure your applications. You'll master:
- How to identify different types of XSS vulnerabilities
- Practical implementation of prevention techniques
- Modern defense mechanisms and security headers
- Step-by-step remediation guidelines
Let's head over to securing your applications against cross-site scripting attacks.
Understanding XSS Attack Vectors
XSS (cross-site scripting) ranks among the most common web security vulnerabilities today. Our research shows XSS vulnerabilities exist in more than half of all applications. This makes understanding these attacks a vital priority.
Common Entry Points for XSS
Attackers have several favorite spots to inject malicious scripts. The most vulnerable areas in applications include:
- Form fields (login forms, comment sections)
- URL parameters and query strings
- HTTP headers (including Referer and User-Agent)
- File upload features
- Rich text editors
- Error messages that show user input
XSS Attack Patterns and Techniques
XSS attacks come in three main types: Stored XSS, Reflected XSS, and DOM-based XSS. Stored XSS happens when attackers plant malicious scripts permanently on target servers, usually in databases or message forums. Reflected XSS occurs when applications instantly return user input through error messages or search results.
DOM-based XSS stands out because it targets the Document Object Model (DOM) in the victim's browser. This type differs from regular XSS attacks because the malicious script doesn't need server storage or delivery.
Impact Assessment of Different XSS Types
Each type of XSS attack can cause different levels of damage. Stored XSS poses the biggest threat because malicious scripts stay on the server and affect everyone who visits the compromised page.
Our research shows successful XSS attacks can lead to these security breaches:
- Session hijacking through cookie theft
- Capture of user passwords and sensitive data
- Execution of unauthorized transactions
- Installation of malware through browser vulnerabilities
The most worrying aspect is how these attacks can bypass standard security measures like the same-origin policy. Users with privileged access who fall victim to these attacks might unknowingly give attackers full control over application functionality and data.
Identifying XSS Vulnerabilities
XSS vulnerability detection works best with a multi-layered strategy. Our team has learned that automated tools combined with manual testing give us the best coverage to find these vulnerabilities.
Code Review Best Practices
Security assessment starts with a detailed code review. OWASP states that "if code has not been reviewed for security holes, the likelihood that the application has problems is virtually 100%". Our code reviews focus on:
- Identifying code segments that output user input
- Verifying proper input validation mechanisms
- Checking encoding implementations
- Reviewing URL handling procedures
- Analyzing sanitization methods
Automated Security Testing Tools
Automated scanning tools are vital to find XSS vulnerabilities at scale. We rely on these key tools:
- Static Application Security Testing (SAST): Analyzes source code to identify vulnerabilities before deployment
- Dynamic Application Security Testing (DAST): Tests running applications to detect security flaws
- Interactive Application Security Testing (IAST): Combines SAST and DAST approaches for complete coverage
These tools use sophisticated algorithms to analyze web application code and identify potential security flaws. The team answers most security vulnerability support tickets within an hour. This quick response time makes automated tools essential.
Manual Testing Approaches
Manual testing remains irreplaceable despite automation advances. We use both black-box and gray-box testing methods. Black-box testing helps us identify input vectors vulnerable to XSS injection and craft specific payloads to assess their effect.
Gray-box testing lets us analyze source code and sanitization procedures with partial knowledge of the application. This approach helps us:
- Test the application's response to injected payloads
- Review existing security measures' effectiveness
- Understand potential risks of found vulnerabilities
- Create proof-of-concept demonstrations
Manual testing helps us find vulnerabilities that automated tools might miss, especially with complex applications. The combination of automated scanning and thorough manual testing creates the most resilient XSS vulnerability detection strategy.
Implementation-Level Prevention
XSS prevention needs multiple layers of protection. No single technique can provide complete protection against XSS attacks. Let's look at the key strategies that create a complete defense system.
Input Validation Strategies
Input validation serves as our first line of defense. Experience shows that validation must happen on both client and server sides. Here's a proven validation approach that works:
- Define strict input criteria based on expected data formats
- Implement server-side validation as the main defense
- Reject inputs containing unnecessary special characters
- Use regular expressions to enforce specific patterns
- Apply length restrictions to prevent buffer overflows
Output Encoding Methods
Output encoding is the foundation of preventing XSS vulnerabilities. Each context needs its own encoding method:
- HTML Context: Convert special characters to HTML entities
- < becomes <
- becomes >
- & becomes &
- JavaScript Context: Unicode-escape non-alphanumeric values
- < becomes \u003c
- becomes \u003e
- CSS Context: Encode values for CSS properties
- URL Context: Apply URL encoding for parameters
The timing of encoding is vital - it should happen right before user-controllable data goes to a page. The context determines what type of encoding you need.
Framework-Specific Security Features
Modern web frameworks come with built-in XSS protection. Applications using these frameworks tend to have fewer XSS vulnerabilities. Developers should know how their framework's security features work:
React DOM automatically escapes all output by default. This gives great protection, but you need to watch out for features like dangerouslySetInnerHTML
.
Angular handles automatic output encoding. Be careful with bypass functions like bypassSecurityTrustAs*
.
These tips help when using frameworks:
- Learn about built-in security mechanisms
- Find potential security gaps
- Use framework-specific encoding functions
- Keep framework components up to date
Note that frameworks offer good protection but aren't perfect. Additional encoding and validation help cover any framework gaps.
Modern XSS Defense Mechanisms
Modern defense mechanisms against cross-site scripting have evolved beyond simple input validation and sanitization. Our team has found that multiple layers of protection provide the most resilient security against sophisticated XSS attacks.
Content Security Policy Implementation
Content Security Policy (CSP) serves as our main defense-in-depth technique to prevent XSS attacks. Traditional host-allowlist approaches often fail and attackers can bypass them. Nonce or hash-based CSP implementations with strict dynamic directives work better.
Our strict CSP includes these essential components:
- Nonce-based script validation
- Hash-based inline script control
- Strict dynamic directive implementation
- Object source restrictions
- Base URI limitations
CSP proves most effective when teams customize it for individual applications rather than using enterprise-wide policies. This method lets us tailor protection based on specific application needs without compromising security.
Security Headers Configuration
Properly configured security headers add an important layer of protection. The X-XSS-Protection header, though supported in older browsers, adds little value in modern browsers that use strong CSP implementations.
Our security headers focus on:
Content-Security-Policy: This serves as our main defense mechanism through nonce or hash-based approaches. It protects better than traditional allowlist-based policies.
X-Content-Type-Options: Setting this to 'nosniff' stops MIME type confusion attacks. This configuration helps prevent specific XSS attacks that target content-type vulnerabilities.
Browser Security Features
Modern browsers offer powerful defensive mechanisms that help reduce potential XSS attack impacts. The Trusted Types API helps prevent DOM-based XSS vulnerabilities by ensuring only secure data types can enter the DOM.
Cookie protection needs special attention in browser security features. The HttpOnly flag on cookies, particularly session cookies, blocks JavaScript access. This measure reduces the impact of successful XSS attacks by protecting sensitive cookie data.
Web Application Firewalls (WAFs) often get suggested as defense mechanisms, but they prove unreliable for XSS attack prevention. Attackers regularly find new bypass techniques, and WAFs miss client-side XSS vulnerabilities. Strong CSP configurations and built-in browser security features work better.
Real-World XSS Remediation
Our web security team has learned that fixing cross-site scripting vulnerabilities needs a step-by-step approach. Most organizations fix XSS vulnerabilities within an hour to reduce exploitation risks.
Step-by-Step Fix Guidelines
These proven steps will help you fix XSS issues:
- Input Sanitization: Use trusted libraries like DOMPurify to clean inputs
- Output Encoding: Use context-specific encoding for HTML, JavaScript, and URLs
- Framework Security: Turn on built-in XSS protections in your framework
- Security Headers: Set up appropriate security headers
- Cookie Protection: Add HttpOnly flag to sensitive cookies
Content sanitization before storage or display substantially reduces XSS risks. But you should know that modifying sanitized content later can undo your security measures.
Testing Validation Methods
We combine automated and manual validation techniques in our testing approach. Tools like OWASP ZAP, Burp Suite, and Acunetix work well to scan web applications for XSS vulnerabilities. A full validation needs:
- Static Analysis: Tools like Checkmarx or Fortify analyze source code
- Dynamic Testing: Automated scanners check live applications
- Manual Review: Code reviews focus on input handling
- Penetration Testing: Ground attack scenarios show real risks
One testing method isn't enough. Regular security audits and penetration testing help teams find system infrastructure vulnerabilities and fix potential risks early.
Post-Implementation Security Checks
Security checks after fixes help ensure everything works. Traditional input validation might not be enough for modern web applications. We look at:
Continuous Monitoring: Automated tools scan applications to find new vulnerabilities. This matters because XSS attacks evolve with new browser features and web technologies.
Framework Updates: Current frameworks and security libraries prevent known XSS vulnerabilities that older versions don't fix.
Security Header Verification: Security headers need proper configuration and testing. This includes Content Security Policy setup and cookie security attributes.
These complete checks help us alleviate XSS vulnerabilities in applications of all sizes. Security needs ongoing code reviews and updates to handle new threats.
Conclusion
XSS (Cross-site scripting) continues to pose major risks to web applications even as we learn more about these vulnerabilities. This piece explores how XSS attacks work through different vectors and what it all means for application security.
Our research shows that XSS prevention works best with multiple defensive layers:
- Full input validation and context-aware output encoding
- Modern security mechanisms like Content Security Policy
- Framework-specific security features
- Continuous monitoring and testing
- Regular security audits and updates
Successful XSS prevention needs a solid grasp of both attack patterns and defense mechanisms. Organizations should move beyond simple input sanitization. They need security strategies that combine automated tools with manual testing approaches.
Note that XSS prevention isn't a one-time fix - it needs constant watchfulness. Strong defenses against evolving XSS threats rely on regular code reviews, security audits, and framework updates. The techniques we discussed here can reduce XSS risks by a lot and better protect applications from these persistent threats.
FAQs
Q1. What makes a web application vulnerable to XSS attacks? Web applications become vulnerable to XSS attacks when they fail to properly sanitize or validate user input before including it in the output. This allows attackers to inject malicious scripts that are then executed by other users' browsers.
Q2. How can developers effectively prevent XSS vulnerabilities? Developers can prevent XSS by implementing multiple layers of defense, including thorough input validation, context-aware output encoding, using Content Security Policy (CSP), leveraging framework-specific security features, and regularly updating and patching their applications.
Q3. What are the different types of XSS attacks? There are three main types of XSS attacks: Stored XSS, where malicious scripts are permanently stored on target servers; Reflected XSS, where user input is immediately returned by the application; and DOM-based XSS, which exploits vulnerabilities in the Document Object Model of the victim's browser.
Q4. What role do security headers play in XSS prevention? Security headers, particularly the Content-Security-Policy header, play a crucial role in XSS prevention. When properly configured, they can restrict the sources of content that a browser is allowed to load, significantly reducing the risk of XSS attacks.
Q5. How can organizations ensure ongoing protection against XSS vulnerabilities? Organizations can maintain protection against XSS by implementing continuous monitoring, conducting regular security audits, performing both automated and manual testing, keeping frameworks and libraries up-to-date, and fostering a security-aware development culture that prioritizes code reviews and secure coding practices.